Column Name Mapping¶
When your application uses custom classes to represent data objects stored in the database, Backendless maps the column names in the database to the fields/properties declared in a custom class. For example, consider the following class:
namespace com.sample
{
public class Person
{
public string name { get; set; }
public int age { get; set; }
}
}
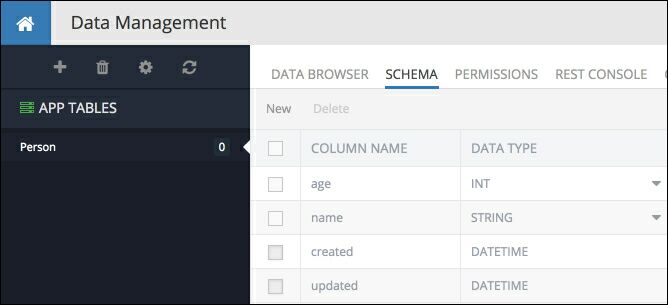
As you can see the names of the columns match the names of the properties in the class. However, sometimes that mapping cannot be observed and a property in the class must have a different name or capitalization than the name of the column. In order to override the default mapping, Backendless supports an attribute which allows to map a property to a column. Consider the following example:
using System;
using Weborb.Service;
namespace com.sample
{
public class Person
{
[SetClientClassMemberName( "name" )]
public string Name { get; set; }
[SetClientClassMemberName( "age" )]
public int Age { get; set; }
}
}
The example demonstrates the mapping of the "Name"
and "Age"
properties in the client application to the "name"
and "age"
columns in the database . The mapping is bidirectional, it means it works for both saving objects in and retrieving from the database.
Important
Creating mappings for system level columns such as objectId, created, updated and ownerId is not supported.