Column Name Mapping¶
When your application uses custom classes to represent data objects stored in the database, Backendless maps the column names in the database to the fields/properties declared in a custom class. For example, consider the following class:
package com.sample;
public class Person
{
public String objectId;
public String name;
public int age;
}
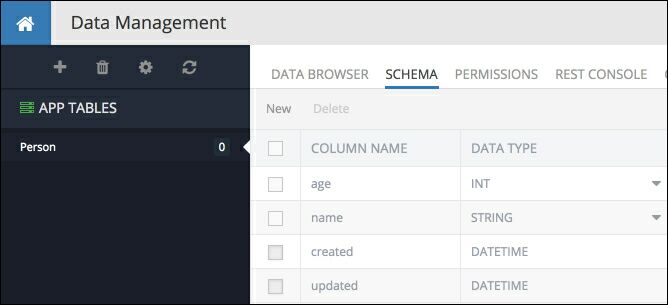
As you can see the names of the columns match the names of the fields in the class. However, sometimes that mapping cannot be observed and a field in the class must have a different name or capitalization than the name of the column. In order to override the default mapping, Backendless supports an annotation which allows to map a public field to a column. Consider the following example:
package com.sample;
import weborb.service.MapToProperty;
public class Person
{
public String objectId;
@MapToProperty( property = "name" )
public String Name;
@MapToProperty( property = "age" )
public int Age;
}
The example demonstrates the mapping of the "Name"
and "Age"
fields in the client application to the "name"
and "age"
columns in the database . The mapping is bidirectional, it means it works for both saving objects in and retrieving from the database.
Important
Creating mappings for system level columns such as objectId, created, updated and ownerId is not supported.
Your class may not always declare public fields. Alternatively, in your implementation you may opt for private field declaration and public getter/setter methods. The annotation is applicable to the methods as well. Consider the example below:
package com.sample;
import weborb.service.MapToProperty;
public class Person
{
private String objectId;
private String Name;
private int Age;
@MapToProperty( property = "age" )
public int getAge()
{
return Age;
}
public void setAge( int age )
{
this.Age = age;
}
@MapToProperty( property = "name" )
public String getName()
{
return Name;
}
public void setName( String name )
{
this.Name = name;
}
public String getObjectId()
{
return objectId;
}
public void setObjectId( String objectId )
{
this.objectId = objectId;
}
}