Extend
This method extends the image to the specified direction and fills the extended areas of the image with the specified color. This feature comes in handy when you have a portrait-oriented image, and you need to extend it to change orientation from portrait to landscape.
In the example below, you can see the same picture before and after the invocation. 200 green pixels were added to the top and right side of the produced image to highlight regions where changes were applied. Note, that the image doesn’t shrink, and only the requested number of pixels are added to the specified sides of the image.
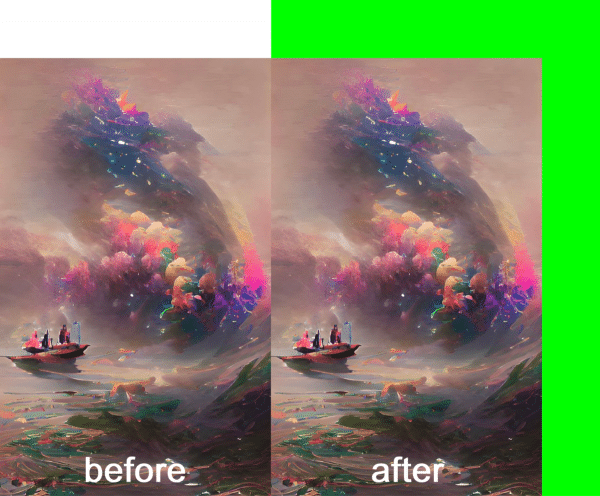
Parameters:
There are 4 direction parameters that accept the integer value which represents the number of pixels to add:
top – The top side of the image is extended by the value passed to this argument. The value is the number of pixels to add.
bottom – The bottom side of the image is extended by the value passed to this argument. The value is the number of pixels to add.
left – The left side of the image is extended by the value passed to this argument. The value is the number of pixels to add.
right – The right side of the image is extended by the value passed to this argument. The value is the number of pixels to add.
background – is an optional parameter consisting of four properties:
r – An integer value in the range between 0 and 255. This parameter identifies the red color component of the final background color.
g – An integer value in the range between 0 and 255. This parameter identifies the green color component of the final background color.
b – An integer value in the range between 0 and 255. This parameter identifies the blue color component of the final background color.
The background parameter is useful, when you need to fill the extended areas with a specific color.
alpha – A double value in the range between 0 and 1. The alpha channel determines the degree of the color opacity for RGB components. Note, that the alpha channel has only one decimal place. The alpha value determines how saturated the requested color is for the extended areas that we specify in the background parameters. The maximum saturation level for the alpha channel is 1, and the minimum level is 0.
If you apply the alpha channel to a .jpg image and the alpha value is 0, the background color becomes black regardless of the RGB profile. In case of a .png image and the alpha channel value set to 0, then the extended area becomes fully transparent.
REST example:
In the example below, the top and right side of the image are extended by 200 pixels. The RGB profile (0,255,0) is applied to the background of the extended areas. The image to modify is located in /image_storage/ai_generated_art.jpg
. After the operation, the modified image is saved to the /folder_to_save_processed_images
.
The method returns a cloud path and the URL to the modified image. If you want to return a redirect URL, the redirect parameter must be set to true.
curl -X "GET" "https://xxxx.backendless.app/api/services/ImageProcessor/extend?image_path=/image_storage/ai_generated_art.jpg&top=200&right=200&background={"r":0,"g":255,"b":0}" \
-H 'Content-Type: application/json' \
-H 'Accept: application/json'
Codeless block example:
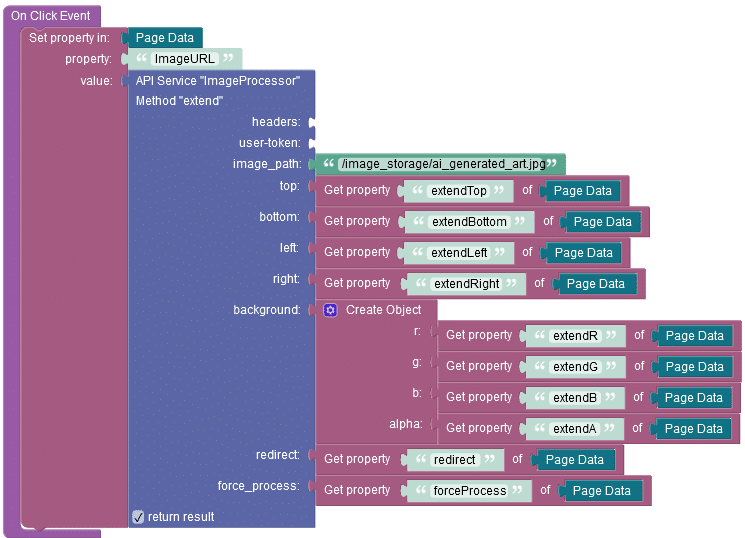