API
User Registration
To enable the 2FA for a user, use the Registration API and pass the totpAuthEnabled
property with the value of true
. The registration API returns an object that contains the following properties:
transactionId
– String value. It must be used in a subsequent call to login the user.
qrURL
– String value. Contains a URL to the QR code that is unique for the registering user. Your application can present the QR code so the user can activate their TOTP app.
secret
– String value. Your application can present this value so the user can activate their TOTP app. The secret
value and qrURL
are alternative ways to achieve the same result – registering your app in the TOTP app.
The Codeless logic below illustrates the usage of the API:
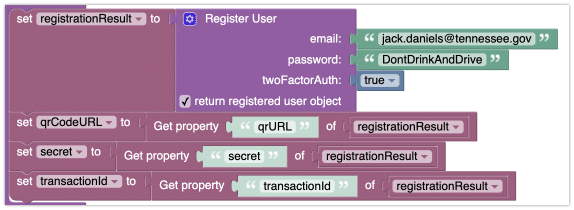
After the user is registered, your application should present the QR and/or secret code to the user and ask them to enter a TOTP password. You need to complete the process by using the following Codeless logic:
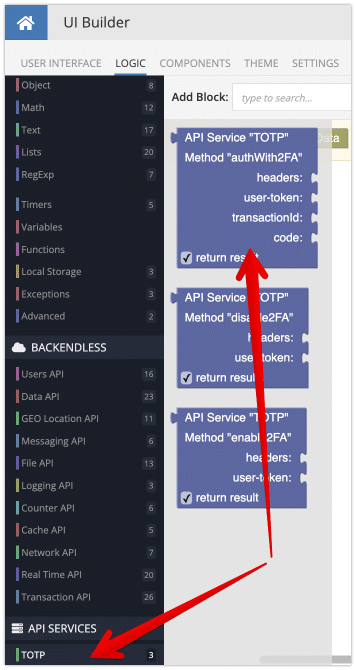
The authWith2FA
method from the TOTP service should be used as shown below. Notice the result of the authWith2FA
method is used in the Set Current User block.
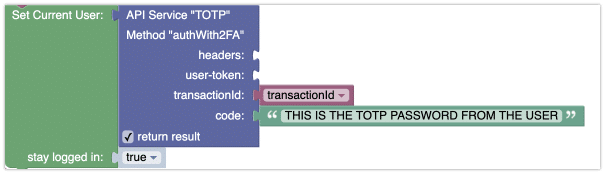
If you are using code, the functionality is available in all Backendless SDKs. Since the API is very similar if not identical between the SDKs, below is a reference with JavaScript:
const { transactionId, qrURL, secret } = await Backendless.Users.register({
email: 'jack.daniels@tennessee.gov',
password: 'DontDrinkAndDrive',
totpAuthEnabled: true })
// present the QR code and the secret value to the user,
// and provide a form for them to enter a password from a TOTP app.
// Once you receive the TOTP password from the user, make the following request:
const code = '123456' // received from a TOTP app (Google Authenticator or Twilio Authy)
const loggedInUser = await Backendless.CustomServices.invoke('TOTP', 'authWith2FA', {
transactionId,
code
})
// set the received user to the SDK
User Login
The 2FA Login process consists of two steps:
- Use the Backendless Login API with the user’s userId (email) and password. If 2FA is enabled for the user account (this is done by setting the
totpAuthEnabled
parameter to true
in the registration API shown above or by the API described below), then the Login API returns transactionId
which should be used in step 2:
- Invoke a method from the TOTP service to finalize the login. The method accepts
transactionId
from the first step and a TOTP password obtained from the user.
Below is the Codeless implementation of the workflow:

After the login screen, if you obtained transactionId
without any errors, present a screen where the user can provide a TOTP password. After that, use the following codeless block to complete the login:
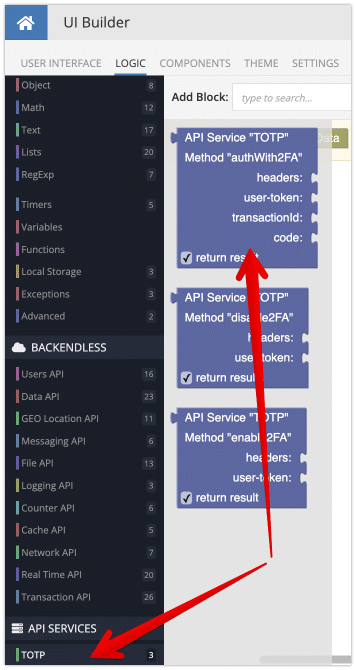
The authWith2FA
method from the TOTP service should be used as shown below. Notice the result of the authWith2FA
method is used in the Set Current User block.
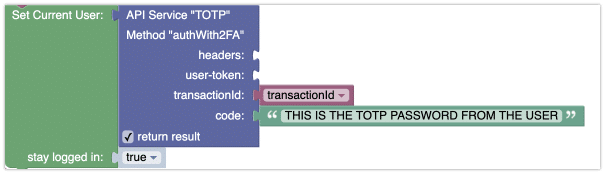
If you are using code, the functionality is available in all Backendless SDKs. Since the API is very similar if not identical between the SDKs, below is a reference with JavaScript:
const { transactionId } = await Backendless.Users.login('jack.daniels@tennessee.gov', 'my-password')
const code = '123456' // TOTP password received from Google Authenticator or Twilio Authy app (or any other TOTP app)
const loggedInUser = await Backendless.CustomServices.invoke('TOTP', 'authWith2FA', {
transactionId,
code
})
// set the received user to the SDK
Enable/Disable 2FA
Since 2FA can be configured at the individual user account level, your application can offer to turn on and off the 2FA functionality to your users. When a user decided to enable or disable that functionality, you should use the API documented below. The APIs require that there is a logged-in user in your application. If there is no logged-in user, and you use the API, the server will return an error.
The following Codeless blocks provide the functionality to enable or disable 2FA for the currently logged in user:
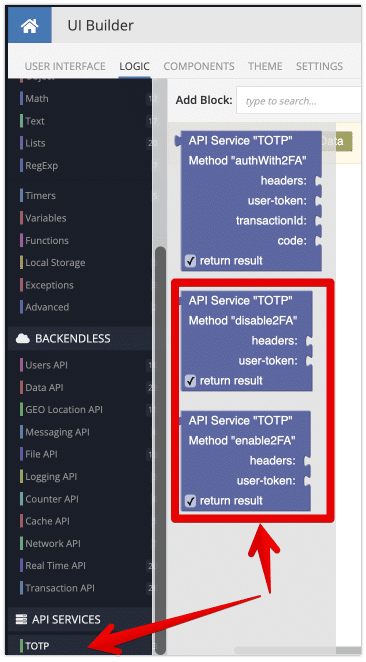
The enable2FA
block returns a QR code URL and secret value that your app may present to the user so they can onboard their TOTP app:
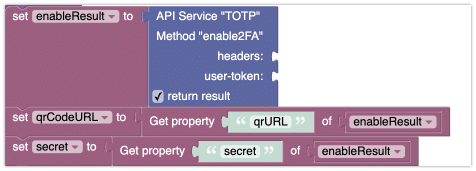
If you are using code, the functionality is available in all Backendless SDKs. Since the API is very similar if not identical between the SDKs, below is a reference with JavaScript:
Enable 2FA:
// NOTE: this request is intended to be made by a logged in user. If user is not logged in, an error will be thrown
const { qrURL, secret } = await Backendless.CustomServices.invoke('TOTP', 'enable2FA')
// show the QR code and secret key to the user
Disable 2FA:
// NOTE: this request is intended to be made by logged in user. If user is not logged in, an error will be thrown
await Backendless.CustomServices.invoke('TOTP', 'disable2FA')