Sign in with Apple is the fast, easy, and more private way to sign into apps and websites using the Apple ID that you already have. Since Apple released the ability to login with Apple ID, developers are encouraged to implement this solution wherever they already offer other third-party sign-in ways (e.g. Google, Facebook, Twitter, etc.). Apple guarantees security and privacy and even allows you to hide your email address by using Apple’s email relay service – it creates and shares a unique and random email address that forwards to your personal email.
To use the Sign In with Apple plugin, you’ll need:
- an Apple ID that uses 2-factor authentication
- to be signed in to iCloud with this Apple ID on your Apple device
Sign in with Apple is available on Apple devices with the latest software (iOS 13 or later, iPadOS 13 or later, watchOS 6 or later, macOS Catalina 10.15 or later, and tvOS 13 or later) and with participating websites in Safari. You can also use Sign in with Apple with other web browsers and on other platforms, like Android or Windows.
Using the API
The API supported by the Login with Apple plugin can be used either via REST or using a Backendless SDK. To use the API with an SDK, generate the client-side library for the API as shown below:
- Click the Business Logic icon in Backendless Console and select the AppleAuth API service.
- Click the Download Client SDK icon as shown below (the icon is shown in bright green color):
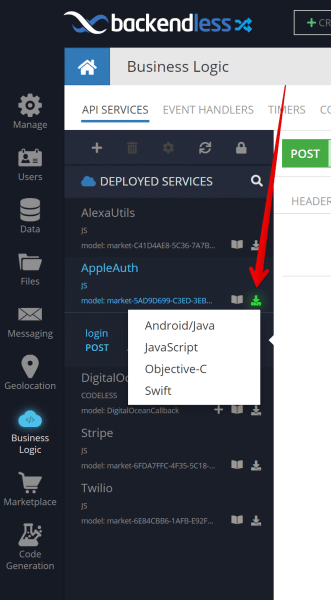
- Select the language of your choice to download the client SDK generated specifically for the AppleAuth service. The SDK includes all the methods provided by the Login with Apple plugin.
In case a language you would like to use does not show up in the list, you can still use the plugin. The information below describes all available options.
Obtaining the Token
Apple provides documentation for how to configure an application or a web page to handle the Apple user credential:
The identity token is a JSON web token (JWT) that securely communicates information about the user to your app. This JWT is a part of the response object (of type ASAuthorizationAppleIDCredential
for Apple devices) and this is exactly the token needed as an argument for the AppleAuth API service’ login
method.
API
Illustrated in the example code below, your application can allow user sign in with Apple using Swift, Obj-C, REST, and JS APIs.
When a token is obtained (per the instructions above), the following API should be used to complete the login:
// with Swift Client SDK - downloaded from Backendless Console per the instructions above
if let appleIdCredential = authorization.credential as? ASAuthorizationAppleIDCredential,
let identityToken = appleIdCredential.identityToken,
let tokenString = String(data: identityToken, encoding: .utf8) {
// login in Backendless using Apple user token
AppleAuth.shared.login(token: tokenString, responseHandler: { loggedInUser in
Backendless.shared.userService.currentUser = loggedInUser
print("User has been logged in using Apple Sign In")
}, errorHandler: { fault in
print("Error: \(fault.message ?? "")")
})
}
// with Swift-SDK
if let appleIdCredential = authorization.credential as? ASAuthorizationAppleIDCredential,
let identityToken = appleIdCredential.identityToken,
let tokenString = String(data: identityToken, encoding: .utf8) {
// login in Backendless using Apple user token
Backendless.shared.customService.invoke(
serviceName: "AppleAuth",
method: "login",
parameters: tokenString,
responseHandler: { loggedInUser in
Backendless.shared.userService.currentUser = loggedInUser
print("User has been logged in using Apple Sign In")
},
errorHandler: { fault in
print("Error: \(fault.message ?? "")")
})
}
// with Swift Client SDK - downloaded from Backendless Console per the instructions above
ASAuthorizationAppleIDCredential *appleIdCredential = authorization.credential;
NSData *identityToken = appleIdCredential.identityToken;
NSString *tokenString = [[NSString alloc] initWithData:identityToken encoding:NSUTF8StringEncoding];
if (tokenString != nil) {
// login in Backendless using Apple user token
[AppleAuth.sharedInstance login:tokenString responseHandler:^(BackendlessUser *loggedInUser) {
Backendless.shared.userService.currentUser = loggedInUser;
NSLog(@"User has been logged in using Apple Sign In");
} errorHandler:^(Fault *fault) {
NSLog(@"Error: %@", fault.message);
}];
}
// with Swift-SDK
ASAuthorizationAppleIDCredential *appleIdCredential = authorization.credential;
NSData *identityToken = appleIdCredential.identityToken;
NSString *tokenString = [[NSString alloc] initWithData:identityToken encoding:NSUTF8StringEncoding];
if (tokenString != nil) {
// login in Backendless using Apple user token
[Backendless.shared.customService
invokeWithServiceName:@"AppleAuth"
method:@"login"
parameters:tokenString
responseHandler:^(BackendlessUser *loggedInUser) {
Backendless.shared.userService.currentUser = loggedInUser;
NSLog(@"User has been logged in using Apple Sign In");
} errorHandler:^(Fault *fault) {
NSLog(@"Error: %@", fault.message);
}];
}
POST https://api.backendless.com/services/AppleAuth/login
Request Body is a string value containing the token (must be included into double quotes):
"TOKEN-VALUE"
Response:
{
"userStatus": "ENABLED",
"created": VALUE,
"ownerId": "9693201D-C418-410B-FFAE-427D2D08B800",
"___class": "Users",
"blUserLocale": "en",
"updated": null,
"objectId": "9693201D-C418-410B-FFAE-427D2D08B800",
"user-token" : "VALUE"
}
// with JS Client SDK - downloaded from Backendless Console per the instructions above
const loggedInUser = await AppleAuth.login('TOKEN VALUE')
// with JS-SDK
const loggedInUser = await Backendless.CustomServices.invoke('AppleAuth', 'login', 'TOKEN VALUE')
The result of the AppleAuth API service login
method is a registered BackendlessUser
object that should be set manually in your code as the current user in your application.
Additional information about the Sign in with Apple features can be found at:
https://help.apple.com/developer-account/?lang=en#/devde676e696